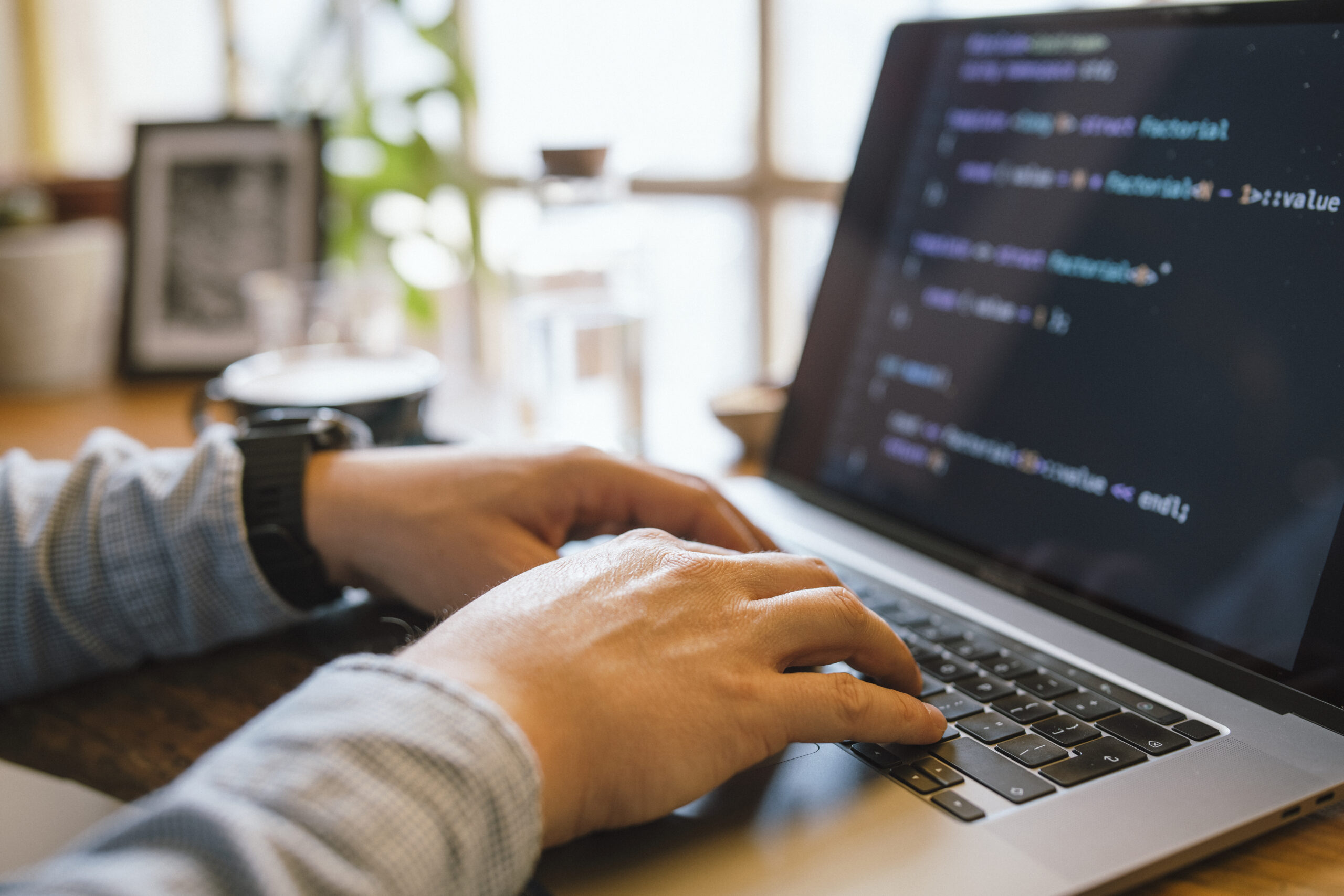
Debugging is one of the most vital — nonetheless often ignored — capabilities in a very developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about understanding how and why things go Incorrect, and Understanding to Feel methodically to resolve difficulties proficiently. No matter whether you're a novice or even a seasoned developer, sharpening your debugging expertise can preserve hours of aggravation and significantly enhance your productivity. Here are several procedures that will help builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Applications
On the list of fastest approaches developers can elevate their debugging skills is by mastering the applications they use on a daily basis. Even though creating code is 1 part of advancement, realizing how you can connect with it proficiently for the duration of execution is equally vital. Modern-day growth environments come Geared up with strong debugging capabilities — but lots of builders only scratch the surface of what these instruments can do.
Choose, by way of example, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the value of variables at runtime, move by means of code line by line, and even modify code to the fly. When utilized the right way, they Allow you to notice precisely how your code behaves all through execution, which can be a must have for tracking down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for front-close developers. They help you inspect the DOM, keep track of community requests, view actual-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can flip discouraging UI issues into manageable jobs.
For backend or system-degree developers, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management around operating processes and memory administration. Discovering these tools might have a steeper Finding out curve but pays off when debugging performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into snug with version Manage programs like Git to be aware of code record, find the exact second bugs had been released, and isolate problematic changes.
In the end, mastering your resources implies heading over and above default options and shortcuts — it’s about establishing an personal familiarity with your progress ecosystem to make sure that when issues crop up, you’re not lost in the dark. The greater you know your applications, the greater time you could expend resolving the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the issue
Probably the most crucial — and often missed — techniques in productive debugging is reproducing the challenge. Just before jumping into the code or earning guesses, builders want to create a dependable natural environment or circumstance in which the bug reliably appears. Devoid of reproducibility, repairing a bug gets to be a game of probability, typically leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as is possible. Request questions like: What steps led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it becomes to isolate the precise circumstances under which the bug happens.
When you’ve gathered sufficient facts, make an effort to recreate the condition in your local ecosystem. This could signify inputting exactly the same facts, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, think about producing automatic tests that replicate the sting conditions or state transitions included. These checks not just enable expose the problem but in addition protect against regressions in the future.
At times, The problem may very well be atmosphere-distinct — it'd happen only on specific running units, browsers, or under certain configurations. Employing applications like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t just a stage — it’s a attitude. It involves tolerance, observation, in addition to a methodical approach. But when you can constantly recreate the bug, you are previously midway to repairing it. By using a reproducible circumstance, You may use your debugging applications extra effectively, test potential fixes safely, and communicate more clearly with your team or users. It turns an summary criticism right into a concrete obstacle — Which’s the place developers thrive.
Study and Comprehend the Mistake Messages
Mistake messages in many cases are the most worthy clues a developer has when anything goes Mistaken. As opposed to viewing them as irritating interruptions, builders really should study to deal with error messages as immediate communications through the program. They frequently tell you what precisely transpired, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Begin by reading the concept very carefully As well as in entire. Several builders, particularly when below time tension, glance at the first line and promptly commence making assumptions. But further within the mistake stack or logs could lie the true root lead to. Don’t just copy and paste mistake messages into search engines like yahoo — read and recognize them initial.
Crack the error down into areas. Is it a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line quantity? What module or purpose triggered it? These inquiries can guide your investigation and position you towards the responsible code.
It’s also handy to know the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally follow predictable designs, and Mastering to recognize these can dramatically increase your debugging course of action.
Some errors are vague or generic, As well as in Those people instances, it’s critical to look at the context in which the error transpired. Test similar log entries, input values, and recent alterations from the codebase.
Don’t overlook compiler or linter warnings either. These usually precede much larger challenges and provide hints about possible bugs.
Eventually, mistake messages are certainly not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Just about the most effective equipment in the developer’s debugging toolkit. When applied proficiently, it offers authentic-time insights into how an software behaves, supporting you fully grasp what’s occurring beneath the hood with no need to pause execution or phase throughout the code line by line.
An excellent logging method begins with understanding what to log and at what level. Common logging levels involve DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts all through progress, Details for basic occasions (like effective start-ups), Alert for likely concerns that don’t break the applying, ERROR for real problems, and Lethal once the system can’t go on.
Prevent flooding your logs with extreme or irrelevant data. Far too much logging can obscure significant messages and slow down your procedure. Center on crucial events, condition alterations, input/output values, and important selection points as part of your code.
Format your log messages Plainly and constantly. Include context, for example timestamps, request IDs, and performance names, so it’s easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all with no halting the program. They’re Specially valuable in creation environments where by stepping by means of code isn’t probable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about harmony and clarity. Which has a nicely-considered-out website logging solution, you'll be able to decrease the time it's going to take to spot concerns, get further visibility into your applications, and Enhance the Over-all maintainability and trustworthiness of your code.
Consider Similar to a Detective
Debugging is not merely a technical activity—it's a sort of investigation. To correctly determine and resolve bugs, builders ought to solution the process like a detective solving a mystery. This attitude will help stop working advanced challenges into workable parts and adhere to clues logically to uncover the root result in.
Commence by collecting evidence. Consider the indicators of the challenge: mistake messages, incorrect output, or effectiveness difficulties. Identical to a detective surveys against the law scene, accumulate just as much suitable facts as you may devoid of leaping to conclusions. Use logs, take a look at situations, and consumer studies to piece with each other a clear picture of what’s going on.
Subsequent, form hypotheses. Ask yourself: What can be producing this behavior? Have any modifications recently been made into the codebase? Has this challenge transpired just before below similar instances? The target is usually to narrow down possibilities and detect probable culprits.
Then, examination your theories systematically. Make an effort to recreate the problem in a managed ecosystem. In case you suspect a particular function or part, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, check with your code queries and Enable the final results lead you nearer to the truth.
Pay near interest to tiny details. Bugs generally conceal during the minimum expected spots—like a lacking semicolon, an off-by-1 mistake, or perhaps a race affliction. Be thorough and client, resisting the urge to patch the issue with no totally knowledge it. Short-term fixes may well hide the true trouble, only for it to resurface later on.
Last of all, maintain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging procedure can preserve time for upcoming concerns and enable others realize your reasoning.
By wondering like a detective, developers can sharpen their analytical capabilities, solution issues methodically, and turn into more practical at uncovering concealed issues in sophisticated programs.
Generate Tests
Creating exams is one of the best solutions to improve your debugging abilities and All round growth effectiveness. Assessments don't just assistance capture bugs early but also serve as a safety net that gives you self-confidence when making changes for your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint specifically in which and when an issue occurs.
Start with unit checks, which focus on individual capabilities or modules. These compact, isolated checks can promptly expose no matter whether a particular piece of logic is Operating as expected. Any time a exam fails, you promptly know the place to seem, substantially decreasing the time used debugging. Device exams are Particularly useful for catching regression bugs—challenges that reappear immediately after Formerly becoming fixed.
Upcoming, integrate integration tests and close-to-conclusion exams into your workflow. These help make sure a variety of elements of your software operate with each other effortlessly. They’re notably beneficial for catching bugs that occur in advanced techniques with multiple parts or solutions interacting. If a little something breaks, your exams can tell you which Section of the pipeline failed and underneath what circumstances.
Producing exams also forces you to definitely Believe critically regarding your code. To test a element correctly, you require to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way prospects to raised code structure and less bugs.
When debugging a difficulty, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails continually, you can target correcting the bug and watch your examination go when The difficulty is resolved. This strategy makes certain that the same bug doesn’t return Later on.
In a nutshell, crafting tests turns debugging from a aggravating guessing video game right into a structured and predictable process—assisting you catch far more bugs, a lot quicker and more reliably.
Get Breaks
When debugging a difficult situation, it’s quick to be immersed in the problem—staring at your display for hours, making an attempt Resolution immediately after Alternative. But Just about the most underrated debugging equipment is actually stepping away. Using breaks will help you reset your brain, lessen annoyance, and sometimes see The problem from the new viewpoint.
When you are also close to the code for as well lengthy, cognitive fatigue sets in. You may start overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain gets to be much less efficient at trouble-resolving. A brief walk, a coffee crack, or maybe switching to a distinct activity for 10–quarter-hour can refresh your emphasis. Several developers report finding the foundation of a difficulty after they've taken time to disconnect, permitting their subconscious operate inside the background.
Breaks also enable avert burnout, Particularly during for a longer period debugging periods. Sitting before a display, mentally trapped, is not merely unproductive but also draining. Stepping absent permits you to return with renewed energy and also a clearer attitude. You may quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you before.
When you’re stuck, a fantastic rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that time to maneuver close to, extend, or do some thing unrelated to code. It may well really feel counterintuitive, In particular below restricted deadlines, but it in fact leads to more rapidly and more practical debugging Over time.
Briefly, taking breaks is just not an indication of weakness—it’s a wise tactic. It gives your brain Place to breathe, increases your perspective, and will help you steer clear of the tunnel vision that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of fixing it.
Master From Every Bug
Every single bug you come upon is more than just a temporary setback—It truly is a possibility to grow as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each one can educate you anything precious if you make an effort to reflect and evaluate what went Mistaken.
Start out by inquiring on your own a handful of key concerns once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with far better procedures like unit screening, code evaluations, or logging? The answers usually reveal blind spots in your workflow or comprehension and make it easier to Make more robust coding practices relocating forward.
Documenting bugs may also be a great habit. Keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll begin to see designs—recurring concerns or frequent errors—that you can proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with the peers may be especially highly effective. No matter whether it’s through a Slack information, a short create-up, or A fast information-sharing session, assisting Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as important portions of your advancement journey. After all, several of the very best builders are not the ones who write excellent code, but those who continually master from their blunders.
Eventually, Every bug you deal with adds a whole new layer to your skill established. So future time you squash a bug, take a minute to replicate—you’ll come away a smarter, additional capable developer as a result of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.